Lua
Renoise API
The #1 most-liked Renoise tool
Reform
Reform is a granular MIDI-editing tool for Renoise. It is the #1 top tool in the Renoise Tool Forums.
Write-Up
The Concept
After the launch of Paddles, the need for a new Renoise tool became apparent; one that would make it quick and intuitive to powerfully fine–tune strums. The idea was inspired by FL Studio's "Strumizer" tool. It offers knobs and sliders to edit timings and velocities. You get realtime visual feedback of your changes to the notes. You can preview the audio by pressing the spacebar. Renoise, in comparison, lacks any such abilities, requiring users to edit notes one–by–one instead.
FL Studio's Strumizer
The Problem
Being a tracker, Renoise represents musical notes as text on a spreadsheet. By default, each cell on the spreadsheet corresponds to a 16th note. To have notes trigger in–between this 16th–note grid, you must type a hexadecimal value 0x00–0x80 into the "delay" column next to the note. This results in an inefficient process for creating strums that are not in sync with the grid's time division, requiring the user to manually type individual hexadecimal values for each note.
Strum editing in Renoise
The Solution
A strum–editing tool for Renoise that would include: a playful UI, intuitive controls, realtime updates to notes, easy preview playback, strumming across pattern boundaries, and more. This would be quite a complex task to achieve in a tracker–based application.
The Development
Though it would take much too long to discuss every challenge and triumph of Reform's development here, we will discuss a few of the noteworthy ones.
Caching
To allow manipulated notes to overflow into other patterns, avoid colliding with other notes, and wrap from the end of the song to the beginning, it was necessary to know pattern lengths, song lengths, note positions, and more. The handing-over of all that data from Renoise's C++ runtime to its Lua API is slow, and Reform demands huge amounts of that data rapidly, hundreds of times per second. To improve performance, the data would be cached Lua–side after retrieving it from the Renoise runtime, and Renoise's Observer–style notifiers would be used to alert Reform when cached data was no longer valid. This resulted in a dramatic increase in performance (over 100x), and was architected in an easy-to-use, modular API.
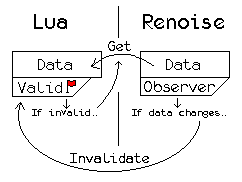
Basic Caching Diagram
Curving
The implementation of Beziér curves in Reform (developed via the Curves demo) allowed for selections of notes to have their timings "bent". Note timings are interpreted as being linearly distributed upon selection, and can then be redistributed along a Quadratic Beziér curve, or an S-shaped Cubic curve. More curve shapes could easily be added, and users could even be allowed to create custom curves via an editor, but for the majority of use–cases, these two curve types are sufficient.
Reform's Curve function in-action
The Result
The tool is accurate, performant, and fully–featured to a professional standard. This was achieved through long–term project organization, disciplined learning, patient problem–solving, and clever optimization. The tool has been provided for free, out of deep appreciation for the Renoise software.
Demonstrating some of Reform's functions